Back in the 1980s, the computing world faced a challenging problem: file systems were a mess. Companies like Apple, IBM and Microsoft – had its own way of organizing files, making sharing data across different platforms quite hard.
Then came the Compact Disc (CD), originally designed for music. However, tech companies quickly realized that CDs could also store software, documents, or operating systems. The problem? There was no universal way to read data CDs across different computers.
Two tech giants, Sony and Philips, worked on the first CD-ROMs, but they needed a file system that would work on any operating system, not just one company’s machines. Enter ISO 9660, a standard created by the International Organization for Standardization (ISO) in 1988.
ISO 9660 ensured that a disc burned on one system could be read on another—whether it was MS-DOS, UNIX, or Mac. This was revolutionary because, at the time, file systems were completely incompatible between platforms.
Fun Fact
The first version of ISO 9660 had strict rules – filenames could only be 8 characters long, plus a 3-character extension (like .TXT or .EXE). This was a leftover limitation from MS-DOS! Later, extensions like Joliet (by Microsoft) and Rock Ridge (for UNIX) were created to allow longer filenames.
The Idea
Not long ago, I realized attaching an ISO to a VM without access to the vCenter or datastores might be helpful. I was surprised that I hadn’t done this before. It’s time to change that.
As always, when I began considering the workflow design, the user would initially be required to give a VM and the complete path to the ISO file. However, my personal OECD suggested that there might be a better approach since copy/pasting can lead to errors. “Make it bullet-proof,” I reminded myself. This means I need to ask the user for a VM, a datastore and/or the folder where the ISO is stored. Because of that, I want the following to be supported in the custom form:
- Browse datastore/s
- Browse folder/s
- Support the root path if ISO is living in
/
of the datastore - Validate if VM has CD-ROM
Let’s start, shall we?
Get Folder
Typically, there is a specific folder on a datastore where all ISO files are stored (if this isn’t the case, I will address that scenario later). Therefore, hard-coding this path is theoretically possible without prompting the user. However, hard-coding limits the reusability of the code.
If multiple folders contain ISO files, I want to allow the user to select a specific folder. To achieve this, a dedicated action element is required. Why is it specific? Well, because I will utilize it in the custom form input field as an external action.
To get and show a list of folders, there are two steps to take:
- Define a query spec (what exactly I want to search for).
- Use this spec together with the
searchDatastore_Task
method.
The function below shows how to do so. A key point is a new VcFolderFileQuery()
class in createQuery
function, which defines what I will search: folder.
Now, I can use that query in the main
function to browse the datastore, get all folders using the extractFolders
function to get only its names, and return it as an array to show in the dropdown later.
if (!datastore)
return [""];
function createQuery() {
var query = new Array();
query[0] = new VcFolderFileQuery();
return query;
}
function createSearchSpec(query) {
var searchSpec = new VcHostDatastoreBrowserSearchSpec();
searchSpec.query = query;
searchSpec.sortFoldersFirst = true;
return searchSpec;
}
function searchDatastore(datastoreBrowser, datastorePath, searchSpec) {
return datastoreBrowser.searchDatastore_Task(datastorePath, searchSpec);
}
function extractFolders(searchResults) {
var folderPaths = [];
for (var _i = 0, _a = searchResults.file; _i < _a.length; _i++) {
var file = _a[_i];
if (file instanceof VcFolderFileInfo) {
folderPaths.push(file.path);
}
}
return folderPaths;
}
function main(datastore) {
var datastoreBrowser = datastore.browser;
var datastorePath = "[" + datastore.name + "] ";
var query = createQuery();
var searchSpec = createSearchSpec(query);
var task = searchDatastore(datastoreBrowser, datastorePath, searchSpec);
var searchResults = System.getModule("com.vmware.library.vc.basic").vim3WaitTaskEnd(task, false, 1);
var folders = extractFolders(searchResults);
System.log("Folders: " + folders);
return folders;
}
return main(datastore);
getFoldersFromDatastore
function
Get ISOs
When I have a directory containing ISOs, I can gather them all in that directory, just as it was done with the previous folders. However, in this instance, my query specification will look for VcIsoImageFileQuery
class. Everything else remains unchanged.
To enable an ISO search in the root directory of the datastore, I defined a straightforward variable called
datastorePath
. This variable determines whether thefolder
variable has been supplied. If it hasn’t, it will search the root level of the datastore.
I also have to make it a separate action element, because this will be an external action in the custom form.
if (!datastore)
return [""];
function createQuery() {
var query = new Array();
query[0] = new VcIsoImageFileQuery();
return query;
}
function createSearchSpec(query) {
var searchSpec = new VcHostDatastoreBrowserSearchSpec();
searchSpec.query = query;
searchSpec.details = new VcFileQueryFlags();
searchSpec.details.fileSize = true;
searchSpec.details.fileOwner = true;
searchSpec.details.modification = true;
searchSpec.details.fileType = true;
searchSpec.searchCaseInsensitive = true;
searchSpec.sortFoldersFirst = true;
return searchSpec;
}
function searchDatastore(datastoreBrowser, datastorePath, searchSpec) {
return datastoreBrowser.searchDatastore_Task(datastorePath, searchSpec);
}
function extractIsoFilePaths(searchResults) {
var isoPaths = [];
for (var _i = 0, _a = searchResults.file; _i < _a.length; _i++) {
var file = _a[_i];
if (file instanceof VcIsoImageFileInfo) {
isoPaths.push(file.path);
}
}
return isoPaths;
}
function main(datastore, folder) {
var datastoreBrowser = datastore.browser;
var datastorePath = "[" + datastore.name + "] ";
if (folder)
datastorePath += folder;
var query = createQuery();
var searchSpec = createSearchSpec(query);
var task = searchDatastore(datastoreBrowser, datastorePath, searchSpec);
var searchResults = System.getModule("com.vmware.library.vc.basic").vim3WaitTaskEnd(task, false, 1);
var isoPaths = extractIsoFilePaths(searchResults);
System.log("ISOs: " + isoPaths);
return isoPaths;
}
return main(datastore, folder);
getISOsFromDatastoreFolder
function
Check if VM has a CD-ROM
To confirm my workflow is highly reliable (something I definitely want to do), it’s essential to evaluate different potential scenarios during execution. One possible option is whether the VM doesn’t have a CD-ROM. Could be? Definitely could. If that’s the case, I should avoid starting the workflow entirely. To achieve this, I should utilize my action element as an external validation function within the custom form.
if (!vm)
return "No VM provided";
var hasCdRom = false;
for (var _i = 0, _a = vm.config.hardware.device; _i < _a.length; _i++) {
var dev = _a[_i];
if (dev instanceof VcVirtualCdrom) {
hasCdRom = true;
break;
}
}
if (!hasCdRom) {
return "VM does not have a CD-ROM";
}
External validation function
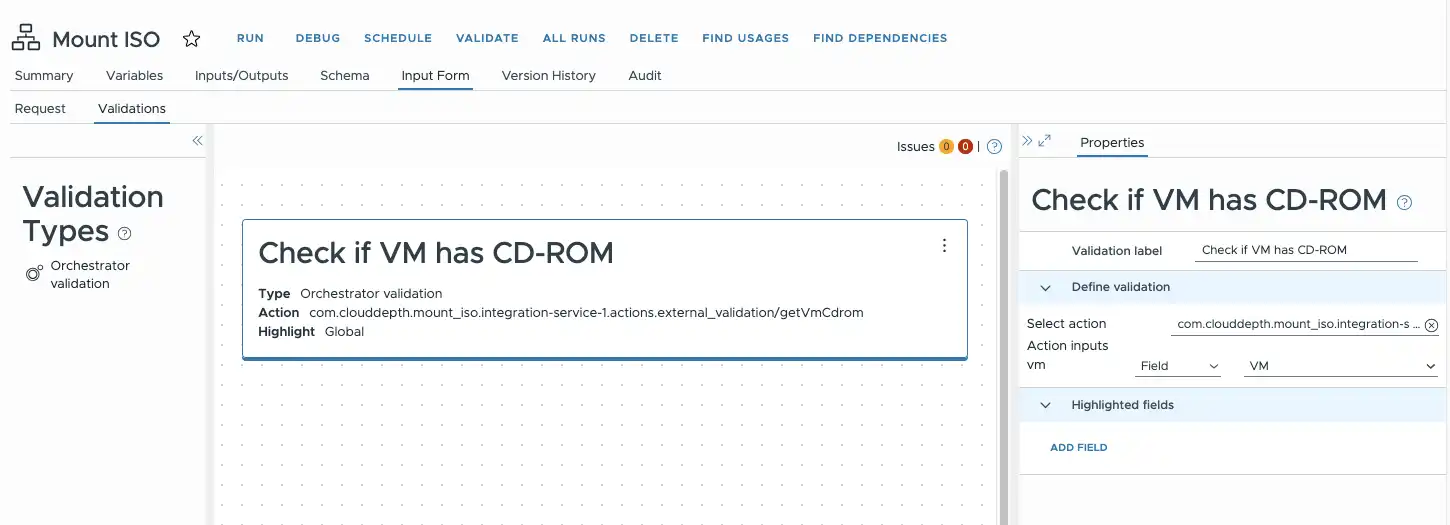
Of course, this example can be expanded even further. For instance, if CD-ROM doesn’t exist, the user can select a checkbox that automatically adds CD-ROM first.
The Workflow
To set up a CD-ROM, I have a function called createCdromDeviceConfig
, which requires specific properties to be defined. One of the parameters I need to supply is the path to the ISO located in cdrom.backing.fileName
.
As seen from that function, more workflow features can be added. For example, I can expose to the user via a custom form a question if ISO should be connected to VM start by providing a boolean to
cdrom.connectable.startConnected
property.
Another essential part is an isoFilePath
variable. The path format vCenter expects to get is [DS NAME] folder/file.iso
. Because of that, I need to construct this path using the variables I am getting from the custom form based on the user selection.
function createCdromDeviceConfig(isoPath) {
var cdromConfig = new VcVirtualDeviceConfigSpec();
var cdrom = new VcVirtualCdrom();
cdrom.connectable = new VcVirtualDeviceConnectInfo();
cdrom.connectable.connected = false;
cdrom.connectable.allowGuestControl = true;
cdrom.connectable.startConnected = true;
cdrom.backing = new VcVirtualCdromIsoBackingInfo();
cdrom.backing.fileName = isoPath;
cdrom.controllerKey = 15000;
cdrom.unitNumber = 0;
cdrom.deviceInfo = new VcDescription();
cdrom.deviceInfo.summary = "Remote ATAPI";
cdrom.deviceInfo.label = "CD/DVD drive 1";
cdrom.key = 16000;
cdromConfig.device = cdrom;
cdromConfig.operation = VcVirtualDeviceConfigSpecOperation.edit;
return cdromConfig;
}
function createReconfigSpec(isoPath) {
var spec = new VcVirtualMachineConfigSpec();
spec.deviceChange = [createCdromDeviceConfig(isoPath)];
return spec;
}
function reconfigureVm(vm, isoPath) {
var spec = createReconfigSpec(isoPath);
System.log("Reconfiguring the virtual machine to mount '".concat(isoPath, "'..."));
try {
var task = vm.reconfigVM_Task(spec);
System.getModule("com.vmware.library.vc.basic").vim3WaitTaskEnd(task, true, 2);
}
catch (error) {
throw new Error("Failed to reconfigure VM: " + error);
}
}
var isoFilePath = "[".concat(datastore.name, "] ").concat(folder, "/").concat(iso);
reconfigureVm(vm, isoFilePath);
Workflows scriptable task
Tests
I need to provide VM and datastore. Once datastore is selected, I can see the list of available folders on that datastore in the Folder field. Let’s pick ISO.
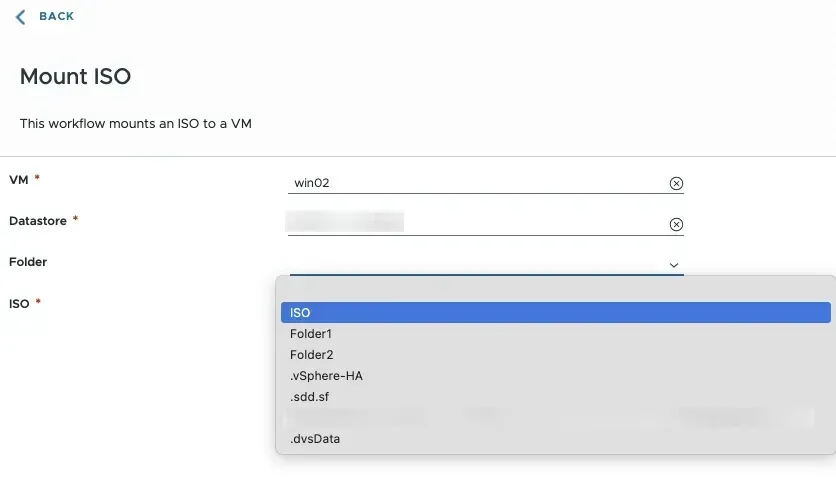
In my ISO folder, there are only two .iso files.
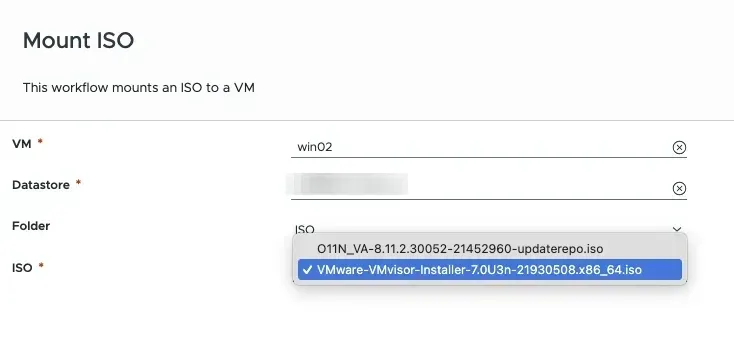
Let’s select O11N and run the workflow.
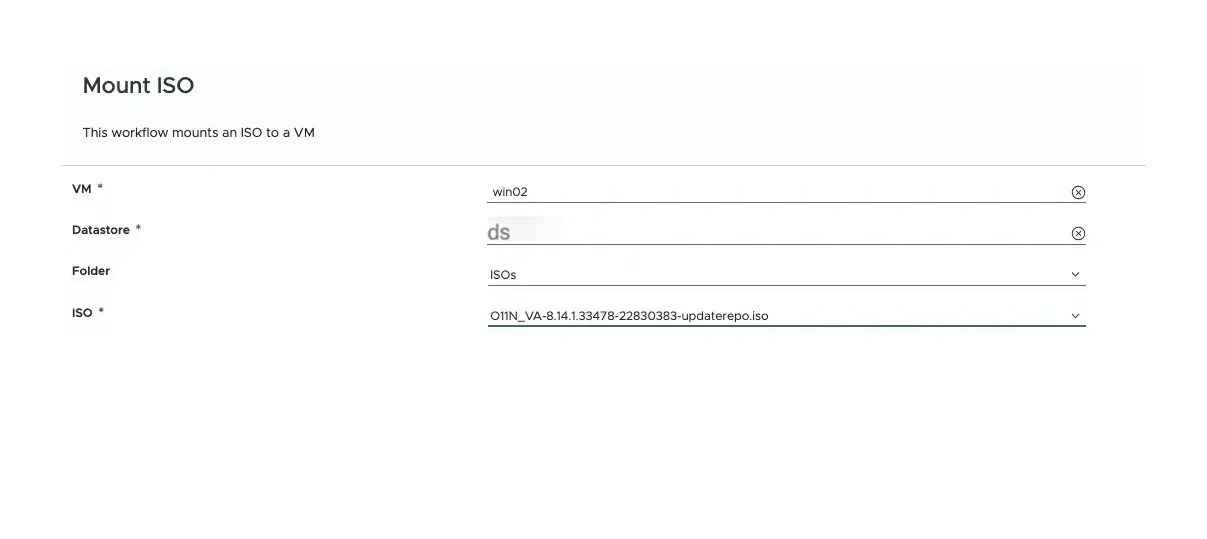
Execution was completed successfully.
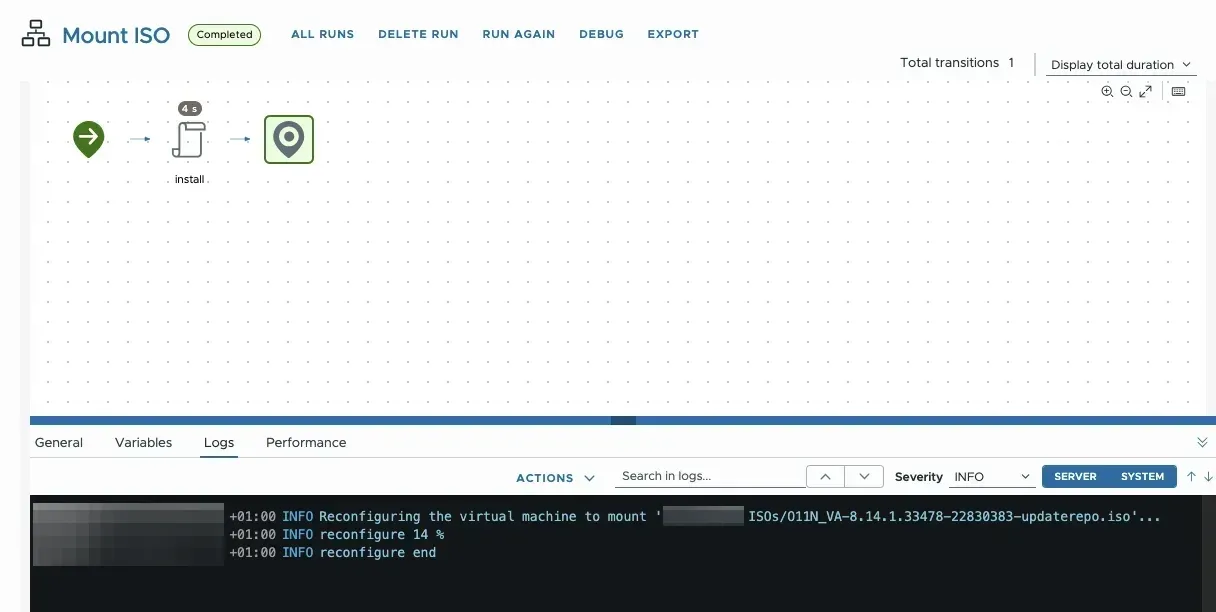
Now let’s take a look at the VM. In the CD/DVD drive section, I can see my ISO is mounted.
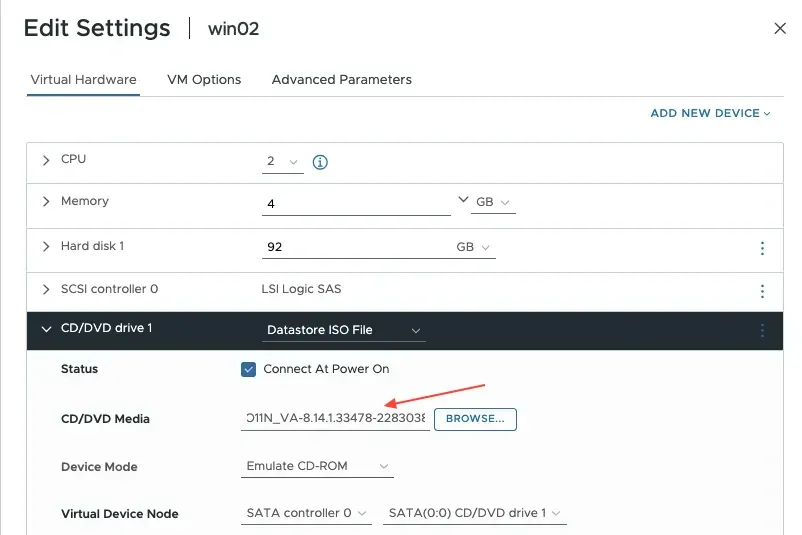
Test without selecting a folder
I can do exactly the same, but without specifying a folder (if I know the ISO is located at the root of the datastore). And, I can see my ISO as well
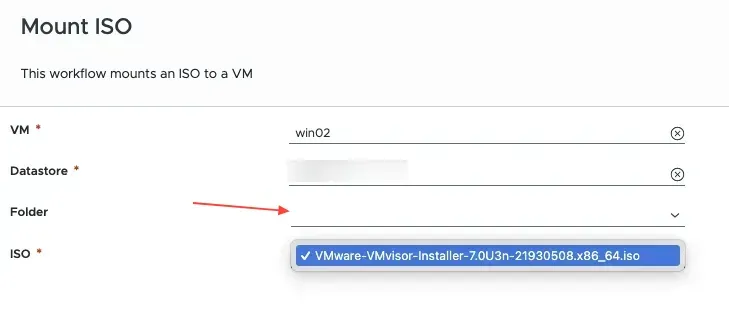
Test without CD-ROM drive
Now I am going to test the workflow’s behavior configuring VM without a CD-ROM. As expected, external validation action did a job and gave me a message clearly saying what the problem is.
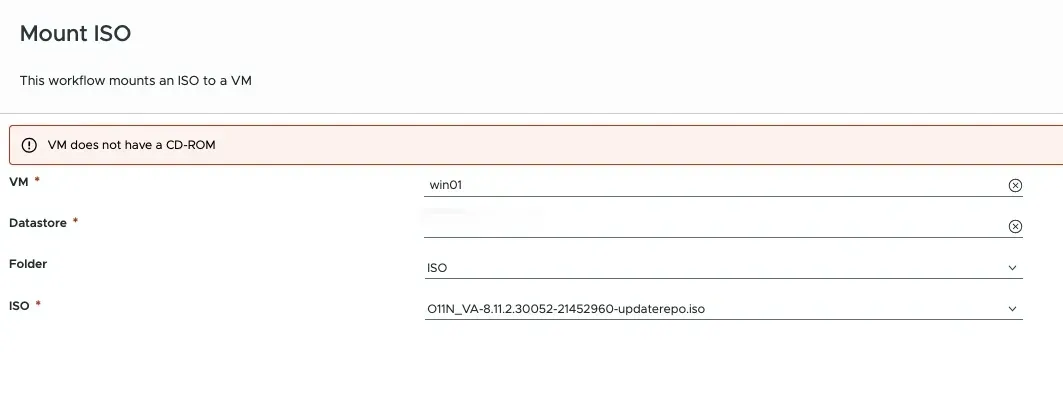
Note that the Folder input isn’t required since I don’t need to indicate a folder. There is no red asterisk next to it.
Tips
When we’re working with custom forms, it’s important to know that specific actions depend on inputs, which can impact the results we get. In a custom form, these inputs can either be fixed values or connected to other fields in the form. Fixed values are simple and safe because if no value is provided, the system just receives an empty string.
However, when we connect action parameters to fields in the form, we need to be more careful. If a field that we’re linking to doesn’t have a default value when the form is first loaded, it will be empty. This can create issues because the action might try to use this empty input, causing the errors.
For example, if a folder
field get its value from datastore
using an action getFoldersFromDatastore
action element, and the datastore
field doesn’t have a value yet, it will cause the action to fail. It is very important to check the existence of the value.
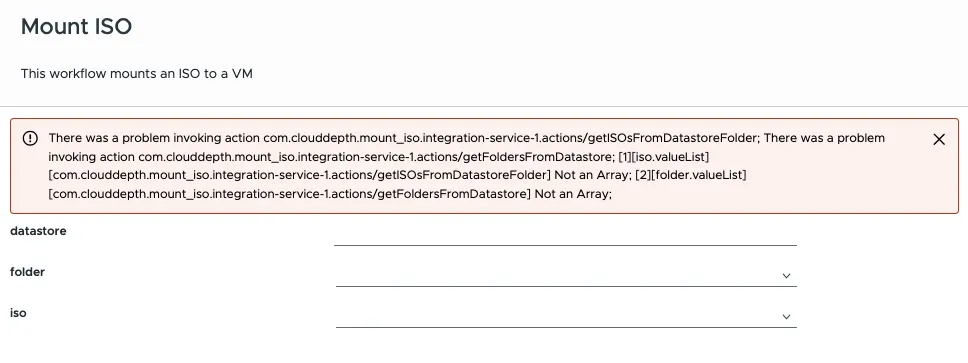
To fix that problem, just add this code at the beginning of the action element. In my example, I added it to the getFoldersFromDatastore
action element.
if (!datastore)
return [""];
This will tell the custom form “show an empty dropdown box” without throwing an error.
Source Code
The source code can be found here.
The vRO package is also available here and the external ECMASCRIPT package here.
All packages should be imported
💡
I also want to hear from you about how I can improve my topics! Please leave a comment with the following:
– Topics you’re interested in for future editions
– Your favorite part or take away from this one
I’ll do my best to read and respond to every single comment!