In the early 1980s, IBM introduced the IBM PC—a modular, relatively open platform that would spark a revolution in personal computing. While IBM expected to dominate the space, they didn’t count on one thing: their design was just open enough to be replicated¹.
Enter Compaq, a company that in 1983 developed a clean-room, reverse-engineered version of IBM’s BIOS². This achievement allowed Compaq to build one of the first fully IBM-compatible PC clones. Soon, dozens of manufacturers entered the scene—Dell, Gateway, HP—all building machines that mimicked the IBM PC’s behavior, especially its BIOS, hardware interrupts, I/O patterns, and memory layout.
This movement became known as the Clone Wars. Clone makers had to ensure their systems were so compatible that software written for the IBM PC would run without modification. If even a single BIOS call or interrupt behaved differently, software like MS-DOS or early versions of Windows would fail to run.
Fast-forward to today, and this story finds a modern parallel in VMware virtual hardware.
Just like the clone PCs of the 1980s, VMware virtual machines are designed to emulate standard x86 hardware. Each VM presents a synthetic set of components: CPUs, disk controllers, NICs, USB interfaces, and more. This virtual hardware is what allows operating systems to boot, install, and operate within a virtual machine just as they would on a physical PC.
VMware maintains virtual hardware versions, each of which represents a generation of virtual devices and capabilities—just like how PC clone generations evolved to support new peripherals and standards³. For example, newer VMware hardware versions might support:
- UEFI firmware instead of legacy BIOS
- NVMe virtual controllers for faster storage
- USB 3.1 virtual ports
This level of emulation ensures maximum compatibility. An OS running in a VMware VM doesn’t need to know it’s virtualized. It just sees standard hardware—much like how MS-DOS saw an IBM PC, whether it was real or a clone.
In a next few minutes, we’ll talk about how to upgrade VM Hardware using JS code.
The idea
One of the recommended steps before upgrading virtual hardware is to ensure that vmtools are updated, up, and running. If so, the hardware can be upgraded.
It is recommended to make a snapshot before, but this article will not cover that part
Check vmtools status
To get the vmtools status, I wrote a simple isVmToolsOk
function. First, I will follow the fail fast best practice.
Essentially, fail fast (a.k.a. fail early) is to code your software such that, when there is a problem, the software fails as soon as and as visibly as possible, rather than trying to proceed in a possibly unstable state.
If a VM object is provided, I can check the status of the tools. If the status is toolsOk
, the function will return true
.
function isVmToolsOk(vm) {
// Ensure the 'vm' parameter is provided and valid
if (!vm) {
throw new Error("Mandatory parameter 'vm' is not defined");
}
var toolsStatus = vm.guest && vm.guest.toolsStatus && vm.guest.toolsStatus.value;
System.log("VMware Tools status: " + toolsStatus);
// Check if the tools status indicates success
return toolsStatus === "toolsOk";
}
Function to check the status of vmtools
Upgrade VM Hardware
To upgrade the VM Hardware, there are a few steps required:
- Get maximum hardware version
- Get current hardware version
- Get vmtools status
Let’s write a primary function that will do all those steps. The name will be helloWorldconfigureVmHardwareUpgrade
.
function configureVmHardwareUpgrade(vm, vmToolsIsUpToDate) {
var maxHWversion = getMaxHWVersion(vm);
var currentHWversion = vm.config.version;
logHWInfo(vm, maxHWversion);
if (isHardwareUpgradeApplicable(vmToolsIsUpToDate, maxHWversion, currentHWversion)) {
System.log("Configuring VM hardware upgrade on next reboot...");
configureHWUpgrade(vm, maxHWversion);
} else {
System.log("Hardware upgrade not possible. vmToolsIsUpToDate: " + vmToolsIsUpToDate + ".");
}
}
Main function
I already did get the vmtools status; therefore, let’s cover the missing functions.
Get maximum hardware version
I don’t want to hardcode anything. Instead, I want to get the maximum version on the fly each time this code is executed. Therefore, there is a simple function that gets it.
function getMaxHWVersion(vm) {
var cods = vm.runtime.host.parent.environmentBrowser.queryConfigOptionDescriptor();
return cods[cods.length - 1].key; // Get the highest supported HW version
}
Get maximum HW version function
Logging
Let’s write a logging function to make my code a bit more informative.
function logHWInfo(vm, maxHWversion) {
System.log("Maximum supported HW version on host " + vm.runtime.host.name + " is: " + maxHWversion);
System.log("Current HW version is: " + vm.config.version);
}
Logging function
Is hardware upgradable
Before starting, I want to make sure the VM Hardware is upgradable. This means all my prerequisites are met: tools are okay, and the maximum hardware version is greater than the current one. To check it, let’s create another function that returns a boolean.
function isHardwareUpgradeApplicable(vmToolsIsUpToDate, maxHWversion, currentHWversion) {
return vmToolsIsUpToDate && maxHWversion > currentHWversion;
}
Is HW upgradeable function
Configure hardware upgrade
Now, I am ready for an upgrade. To do so, I must define a new VcVirtualMachineConfigSpec
class and initialize a VcScheduledHardwareUpgradeInfo
class. There are two properties should be defined:
- upgradePolicy (can be
onSoftPowerOff
,never
oralways
) - versionKey
And yes, let’s make another function 😄.
function configureHWUpgrade(vm, maxHWversion) {
var configSpec = new VcVirtualMachineConfigSpec();
configSpec.scheduledHardwareUpgradeInfo = new VcScheduledHardwareUpgradeInfo();
configSpec.scheduledHardwareUpgradeInfo.upgradePolicy = "onSoftPowerOff";
configSpec.scheduledHardwareUpgradeInfo.versionKey = maxHWversion;
try {
vm.reconfigVM_Task(configSpec);
} catch (e) {
throw new Error("Failed to set hardware upgrade on next reboot. Exception: " + e);
}
}
Configure HW upgrade function
Tests
Create a new workflow. I created a new action element for isVmToolsOk
function. And a scriptable task for the rest of the code.
Execution example: configureVmHardwareUpgrade(vm, vmToolsIsUpToDate)
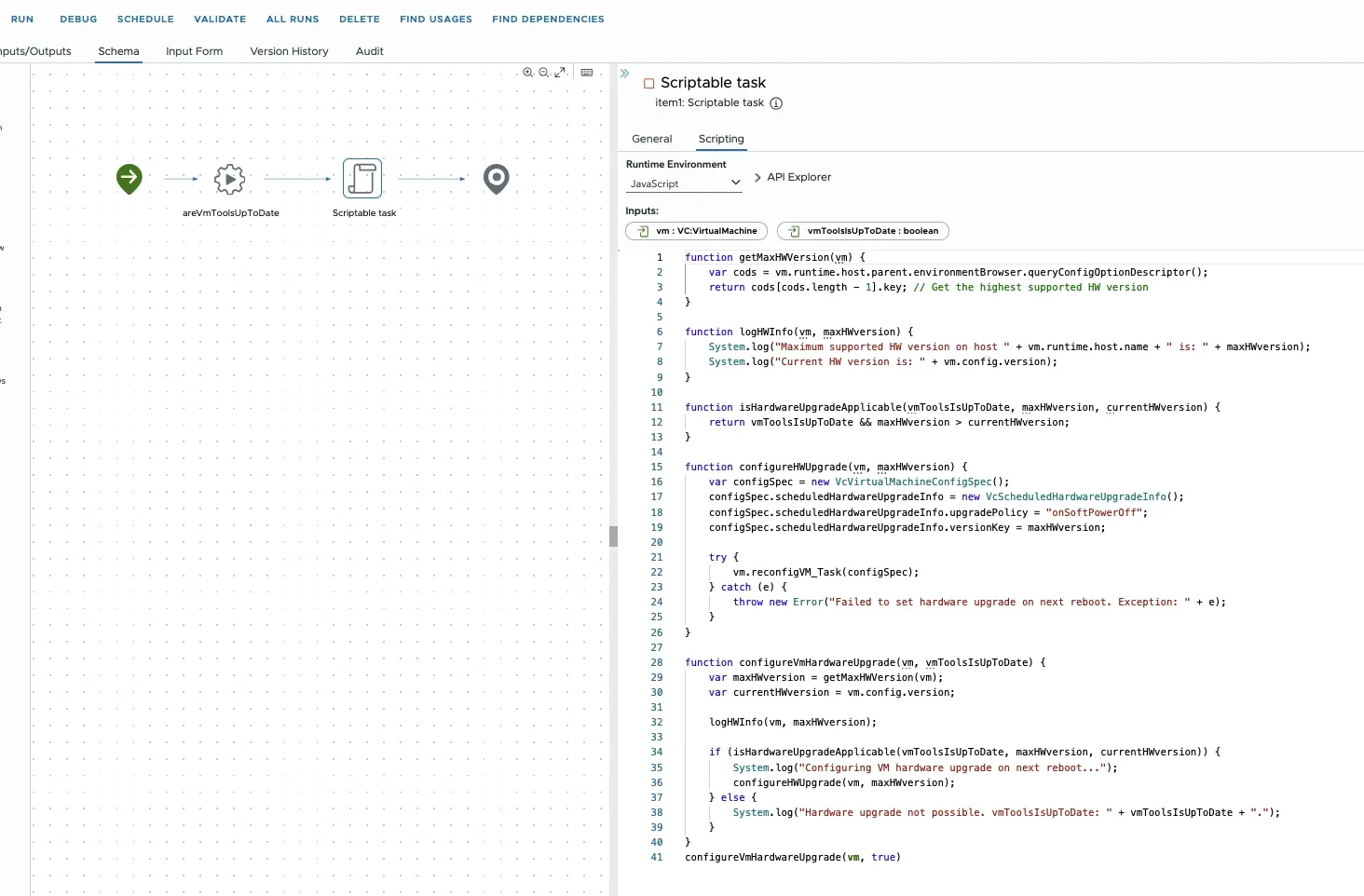

References
- Canion, R. (2013). Open: How Compaq Ended IBM’s PC Domination and Helped Invent Modern Computing. BenBella Books.
- Swaine, M., & Freiberger, P. (2000). Fire in the Valley: The Making of the Personal Computer. McGraw-Hill.
- VMware. (2023). Virtual Machine Compatibility and Virtual Hardware Versions. VMware Knowledge Base. Retrieved from https://kb.vmware.com/s/article/1003746
💡
I also want to hear from you about how I can improve my topics! Please leave a comment with the following:
– Topics you’re interested in for future editions
– Your favorite part or take away from this one
I’ll do my best to read and respond to every single comment!