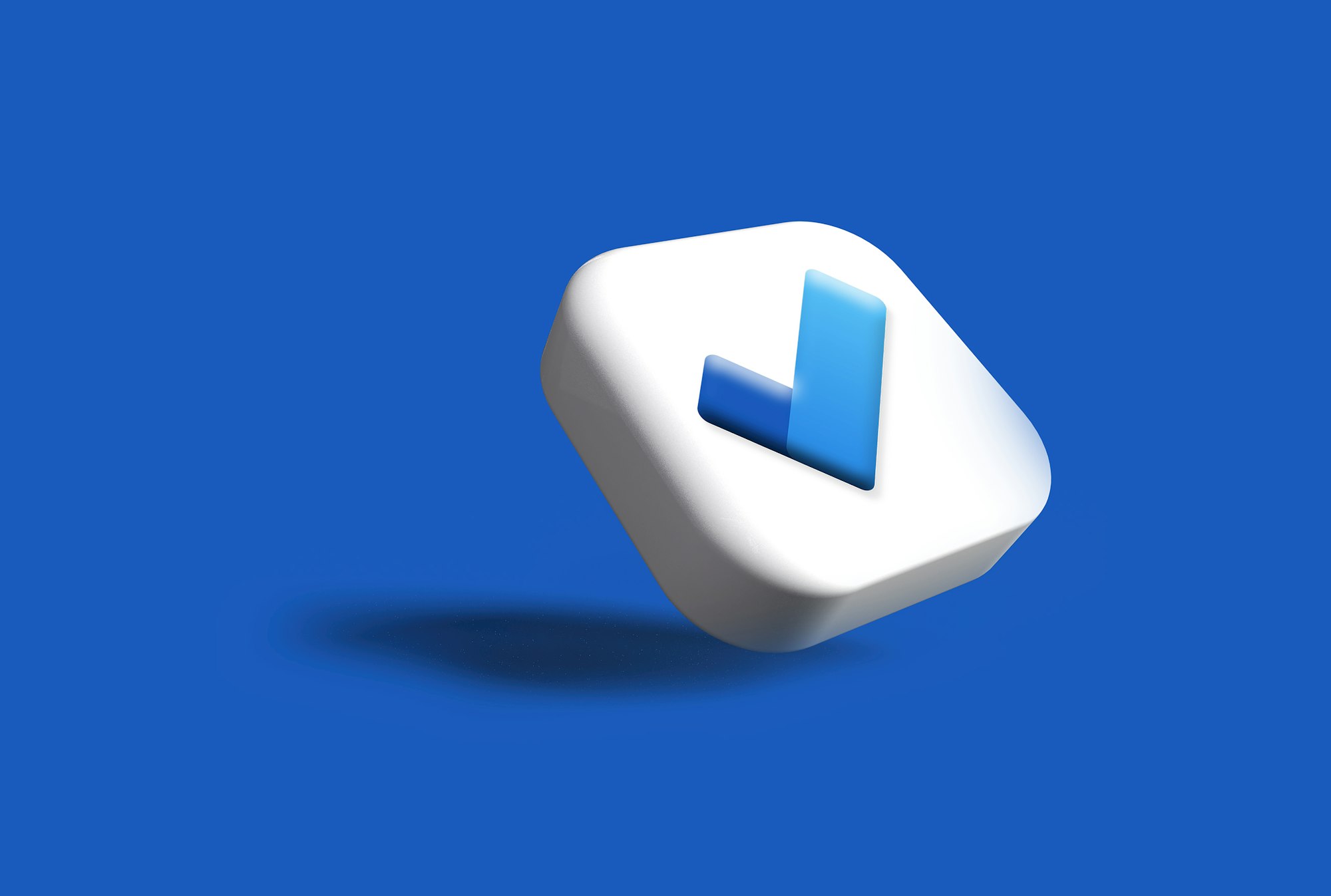
In 1495, Leonardo da Vinci designed what is often considered one of the first robots: an automated knight. While primarily for entertainment, this “mechanical man” could move his arms, sit, and even open his mouth. It may not have swept the floor or prepared dinner, but it shows that the dream of automating tasks is ancient and ever-present.
In a next few minutes, I will show you two basic tasks you can immediately implement and automate your daily routine.
Create new folder in vCenter
It can be a simple action element or scriptable task (an action element is always preferable because this code can be reusable).
As you can see, the only two variables we need are datacenter
and folderName
. Types are noted in JDoc.
/**
* datacenter {VC:DatacenterFolder}
* folderName {string}
*/
function createFolder(datacenter, folderName) {
var folder = datacenter.createFolder(folderName);
System.log("Folder created: " + folder.name);
return folder;
}
// Usage
var folder = createFolder(datacenter, "NewFolderName");
As a result, a new folder called NewFolderName
will be created.
Clone VM
I prefer not to clone VMs (at least production), but there are many use cases where it’s okay. Therefore, let’s take a look at how to do it.
Cloning API requires two classes to be defined:
VcVirtualMachineCloneSpec
VcVirtualMachineRelocateSpec
If the requirement for VcVirtualMachineCloneSpec
class initialization is self-explanatory, the requirement for VcVirtualMachineRelocateSpec
class is not so obvious. The reason for that is one mandatory property: location
. vCenter should know where the source VM should be cloned to. I don’t need to provide a location
if I want the VM cloned to the same place where the source VM lives. I just need to initialize it. This will use, by default, all the same properties of the source VM.
/**
* vm {VC:VirtualMachine}
* folder {VcFolder}
* cloneName {string}
*/
function cloneVM(vm, cloneName, folder) {
var cloneSpec = new VcVirtualMachineCloneSpec();
cloneSpec.powerOn = false;
cloneSpec.location = new VcVirtualMachineRelocateSpec()
var clone = vm.cloneVM_Task(folder, cloneName, cloneSpec);
System.getModule("com.vmware.library.vc.basic").vim3WaitTaskEnd(clone, true, 2)
System.log("Cloning task started for: " + cloneName);
}
// Usage
cloneVM(vm, "CloneVMName", folder);
Summary
Even small reusable functions can significantly ease our workflow, making the time invested in developing them valuable.
💡
I also want to hear from you about how I can improve my topics! Please leave a comment with the following:
– Topics you’re interested in for future editions
– Your favorite part or take away from this one
I’ll do my best to read and respond to every single comment!