In 1901, divers collecting sponges uncovered a shipwreck that dates to the 1st century BCE off the shores of the Greek island of Antikythera. Among the treasures pulled from the seabed was a corroded lump of bronze and wood that initially appeared to be nothing more than debris. However, closer examination revealed a complex system of gears—an astonishing feat of engineering that would come to be known as the Antikythera Mechanism [1].
Built around 100 BCE, the Antikythera Mechanism is now recognized as the world’s oldest known analog computer. It consisted of at least 30 interlocking gears made of bronze, designed to predict celestial events such as the phases of the moon, lunar and solar eclipses, planetary positions, and even the timing of the ancient Olympic Games [2][3]. It implemented the Metonic cycle (19-year lunisolar calendar), and used differential gearing — something not seen again in known mechanical devices for over 1,000 years.
The mechanism’s true complexity remained misunderstood for decades, largely because its intelligence was hidden within layers of corrosion and ancient craftsmanship. It wasn’t until 21st-century imaging techniques like X-ray tomography and 3D CT scanning were applied that researchers finally began to decode the device’s full functionality [4].
Hidden Power in Modern Systems: Just Like VMware ESXi
The Antikythera Mechanism reminds us that sometimes, the most powerful parts of a system are not immediately visible. In the digital world, VMware ESXi works the same way. While its graphical interface exposes a wide array of features, some of the most transformative capabilities lie hidden in its advanced settings, accessible only to those who dig deeper.
In this article, I am going to configure a special place inside the ESXi host called a “Advanced System Settings”. This is like a magic tool that lets you tweak performance, fix tricky problems, and discover hidden powers.
Intro
Advanced Settings in ESXi are key-value parameters that can be updated or deleted. The key is always a string, while the value can be either a string or a number. Even boolean values are represented as strings. The VCF Orchestrator has a dedicated class called VcOptionValue
to work with such settings. I will use it later in my code, but spoiler – it may be tricky to use.
My idea is to create a new, dedicated class called AdvancedSettingsManagement
in the new vcHostSystemManagement.ts
file. As usual, I will create all related classes to host management in this file.
💡
It’s generally a good practice to start a file name (e.g., vcHostSystemManagement.ts
) with lowercase letters. On the other hand, class names (e.g., AdvancedSettingsManagement
) should always begin with uppercase letters.
In my example, I need to update the setting named NFS.MaxVolumes
with a value of 256
. Let’s explore how to accomplish this using VCF Orchestrator.
Inputs validation
As we all know, it’s always a good idea to validate our inputs. So, as usual, I’ll create a simple function to do that.
public validateParameters(key: string, value: any): void {
if (!key) {
throw "Mandatory parameter 'advancedSettingKey' is not defined";
}
if (!value) {
throw "Mandatory parameter 'advancedSettingValue' is not defined";
}
System.log("Advanced setting key: " + key);
System.log("Advanced setting value: " + value);
}
Validate Parameters function
This function simply ensures that both parameters are not empty.
Create Advanced Settings
Next, I’ll write a function called createAdvancedSetting. As mentioned earlier, I need to use a dedicated class to work with advanced settings in ESXi. It can’t be a simple variable. Therefore, I’ll create a new VcOptionValue
class with two main parameters: key and value. Now, here’s the tricky part: the chosen property from the table below will overwrite (and actually work) the type of the variable coming from the outside (for example, from the Variables menu).
Property | Type | Required |
---|---|---|
dynamicProperty | Array/VcDynamicProperty | No |
dynamicType | String | No |
value_IntValue | Number | No |
value_LongValue | Number | No |
value_FloatValue | Number | No |
value_AnyValue | Object | No |
key | String | No |
Let’s examine my example. My value should be 256, which is a number. Therefore, it doesn’t matter if the type of my value in the form will be a string.
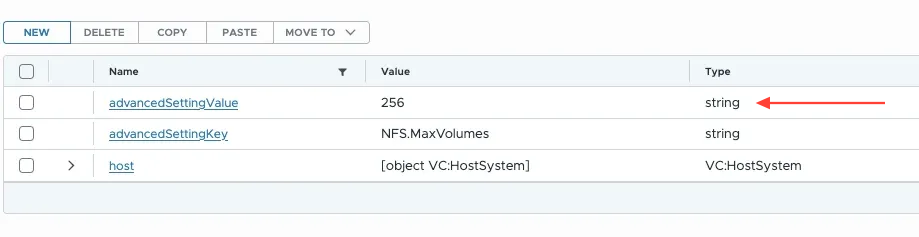
or it will be a Number,
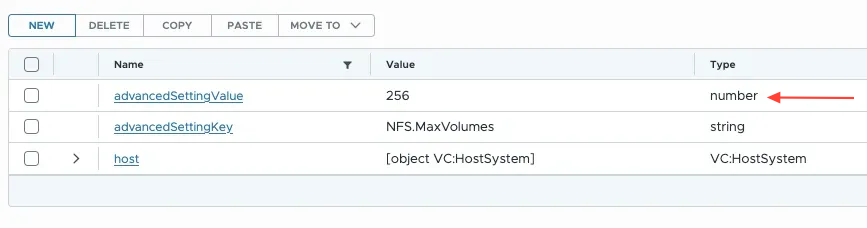
if I want it to work, I must use value_IntValue
in my code.
To make the code bulletproof, I’ll check the variable type and use the appropriate property. This way, I can use properly typed variables in the form.
public createAdvancedSetting(key: string, value: any): VcOptionValue {
let setting: VcOptionValue = new VcOptionValue();
try {
setting.key = key;
if (typeof value === "number") {
setting.value_IntValue = value;
} else {
setting.value_AnyValue = value;
}
return setting;
} catch (e) {
throw "Failed to create advanced setting. " + e;
}
}
Create Advanced Settings function
Apply Advanced Setting
Now, I’m ready to apply my settings. To do this, I need to use the host.configManager.advancedOption.updateOptions
method, which expects an Array
or VcOptionValue
. That’s all.
public applyAdvancedSettingsToHost(host: any, settingsArray: VcOptionValue[]): void {
try {
System.log("Applying advanced settings on host...");
host.configManager.advancedOption.updateOptions(settingsArray);
System.log("Advanced settings have been applied");
} catch (e) {
throw "Failed to add/update host advanced settings. " + e;
}
}
Apply Advanced Settings function
Tests
Let’s create a new workflow with the following inputs or variables.
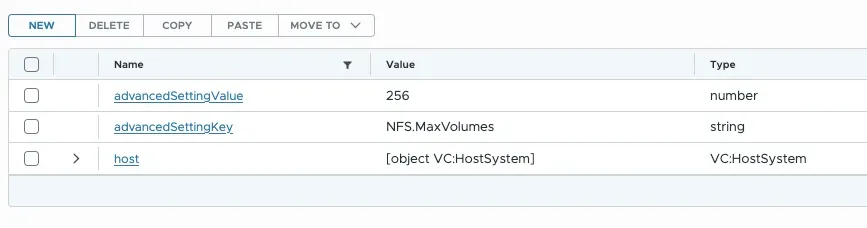
In a scriptable task, I’m calling my AdvancedSettingsManagement
class using vcHostSystemManagement
module. The rest of the process is straightforward.
var hostAdvancedSettings = System.getModule("com.examples.vmware_aria_orchestrator_examples.actions").vcHostSystemManagement();
hostAdvancedSettings.AdvancedSettingsManagement.prototype.logHostInfo(host);
hostAdvancedSettings.AdvancedSettingsManagement.prototype.validateParameters(advancedSettingKey, advancedSettingValue)
var settings = [];
var setting = hostAdvancedSettings.AdvancedSettingsManagement.prototype.createAdvancedSetting(advancedSettingKey, advancedSettingValue)
settings.push(setting);
hostAdvancedSettings.AdvancedSettingsManagement.prototype.applyAdvancedSettingsToHost(host, settings);
Scriptable task code
As a result of the execution, I can see the following in the vRO console log:
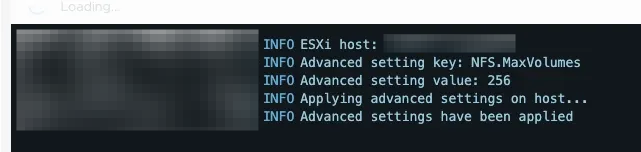
And the updated settings in the vCenter

Summary
As we can see, in most cases, the advanced features are always hidden. Advanced Settings allows to adjust many parameters of ESXi host. Using VCF Orchestrator, we can control and align all of them to the same baseline.
Source Code
The source code with the unit tests can be found here.
The vRO package is also available here and the external ECMASCRIPT package here.
All packages should be imported
📚 References
- Freeth, T., Bitsakis, Y., Moussas, X., et al. (2006). Decoding the ancient Greek astronomical calculator known as the Antikythera Mechanism. Nature, 444(7119), 587–591. DOI: 10.1038/nature05357
- Freeth, T., Jones, A., Steele, J. M., & Bitsakis, Y. (2008). Calendars with Olympiad display and eclipse prediction on the Antikythera Mechanism. Nature, 454(7204), 614–617. DOI:10.1038/nature07130
- Marchant, J. (2010). The ancient computer. Nature, 468, 496–498. DOI: 10.1038/468496a
- Freeth, T. (2014). The Antikythera Mechanism: Decoding an ancient Greek astronomical calculator. Scientific American, 311(6), 76–81. https://doi.org/10.1038/nature05357
💡
I also want to hear from you about how I can improve my topics! Please leave a comment with the following:
– Topics you’re interested in for future editions
– Your favorite part or take away from this one
I’ll do my best to read and respond to every single comment!